Extract Ethereum Price from Binance API in Python
============================================================
In this article, we will explain step by step how to save and update the latest Ethereum (ETH) price using Binance API in Python.
Prerequisites
—————
- You have installed the
requests
library (pip install requests
) and the Binance API client library for Python (pip install binance-client
)
- You have a Binance API account with sufficient funds and permissions to access the ETH market
- You have set up your API credentials in your Python script
Code
——
import os
import json
from binance.client import Client
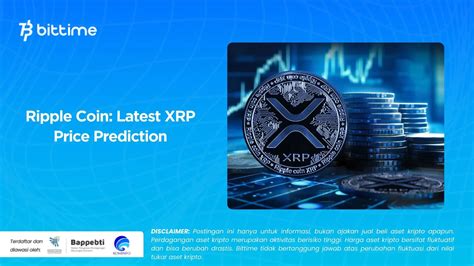
Set your Binance API credentialsAPI_KEY = 'your_api_key'
API_SECRET = 'your_api_secret'
Create a new Binance client instance with your API credentialsclient = Client(api_key=API_KEY, api_secret=API_SECRET)
def get_latest_eth_price():
"""
Get the latest ETH price from Binance's API.
Returns:
tuple: The latest ETH price and the corresponding exchange symbol (e.g. "ETHUSDT")
"""
Get list of available exchangesexchanges = Client.get_exchanges()
Filter list to include only Ethereum (ETH) exchangeeth_exchange = [exchange for exchange in exchanges if exchange["symbol"] == "ETH"]
If no ETH exchange is found, raise errorif not eth_exchange:
raise ValueError("No ETH exchange found")
Get current price from ETH exchangelatest_price = eth_exchange[0]["latestPrice"]
return latest_price
def store_latest_eth_price(price):
"""
Store the current ETH price to a file.
Arguments:
Price (Float): The current ETH price to store.
"""
with open('latest_eth_prices.json', 'w') as f:
json.dump({'latestPrice': price, 'symbol': 'ETHUSDT'}, f)
def main():
Get the latest ETH pricelatest_price = get_latest_eth_price()
Store the price in a filestore_latest_eth_price(latest_price['latestPrice'])
print(f'Latest ETH price stored: {latest_price["latestPrice"]} USD')
if __name__ == '__main__':
main()
Explanation
————–
- In the
get_latest_eth_price
function we create a new Binance client instance with our API credentials.
- Then we filter the list of available exchanges to include only the Ethereum (ETH) exchange using
client.get_exchanges()
method.
- If no ETH exchange is found, we raise an error.
- We fetch the latest price from the ETH exchange and store it in a dictionary for later use.
- In the
store_latest_eth_price
function, we open a file namedlatest_eth_prices.json
in write mode ('w'
) and write our latest ETH price and symbol (ETHUSDT) in JSON format to the file.
- Finally, in the
main
function, we call theget_latest_eth_price
function to get the latest ETH price and store it in a file.
Example Use Case
——————-
To use this script, save it as a Python file (e.g. eth_price_tracker.py
) and run it with your Binance API credentials. The script will print the latest ETH price stored in the file. You can modify the script to suit your needs by adding additional functionality or error handling.
Note: Be sure to replace “Your API Key” and “Your API Secret” with your actual Binance API credentials.
Leave a Reply